How to Use the Mautic API
Are you looking to automate repetitive tasks or integrate Mautic with other platforms? Do you need to create, modify or delete a lot of data and want to use a script you wrote? The Mautic API offers a robust solution to fulfill your needs.
Learn how to enable, use, and secure your Mautic's API functionality and usage. This allows you to integrate 3rd party tools/services or automate tasks with your own scripts.
Mautic's REST API
Mautic comes with a complete RESTful API that lets you interact with almost any part of Mautic.
Just in case you're wondering:
What is an API?
An API, or Application Programming Interface, is essentially a gateway that allows one software program to interact with another.
So, in our case a CRM with Mautic or your script with Mautic.
If you're new to API's the question is: What's it good for? What's in for me?
Let me show you 3 benefits:
Benefits of Using Mautic API
With Mautic's API you can automate your marketing tasks. Here are some examples:
- Automation of Repetitive Tasks: Automate tasks like importing emails from another source, import, update, or edit contacts or contact lists, manipulate contact points or stages based on external triggers or calculations.
- Integration with Other Tools: Connect Mautic seamlessly with CRM platforms, e-commerce systems, or other marketing tools you use.
- Customization Possibilities: Tailor Mautic to your specific marketing needs by building custom integrations or functionality that’s unique to your business.
Use Cases
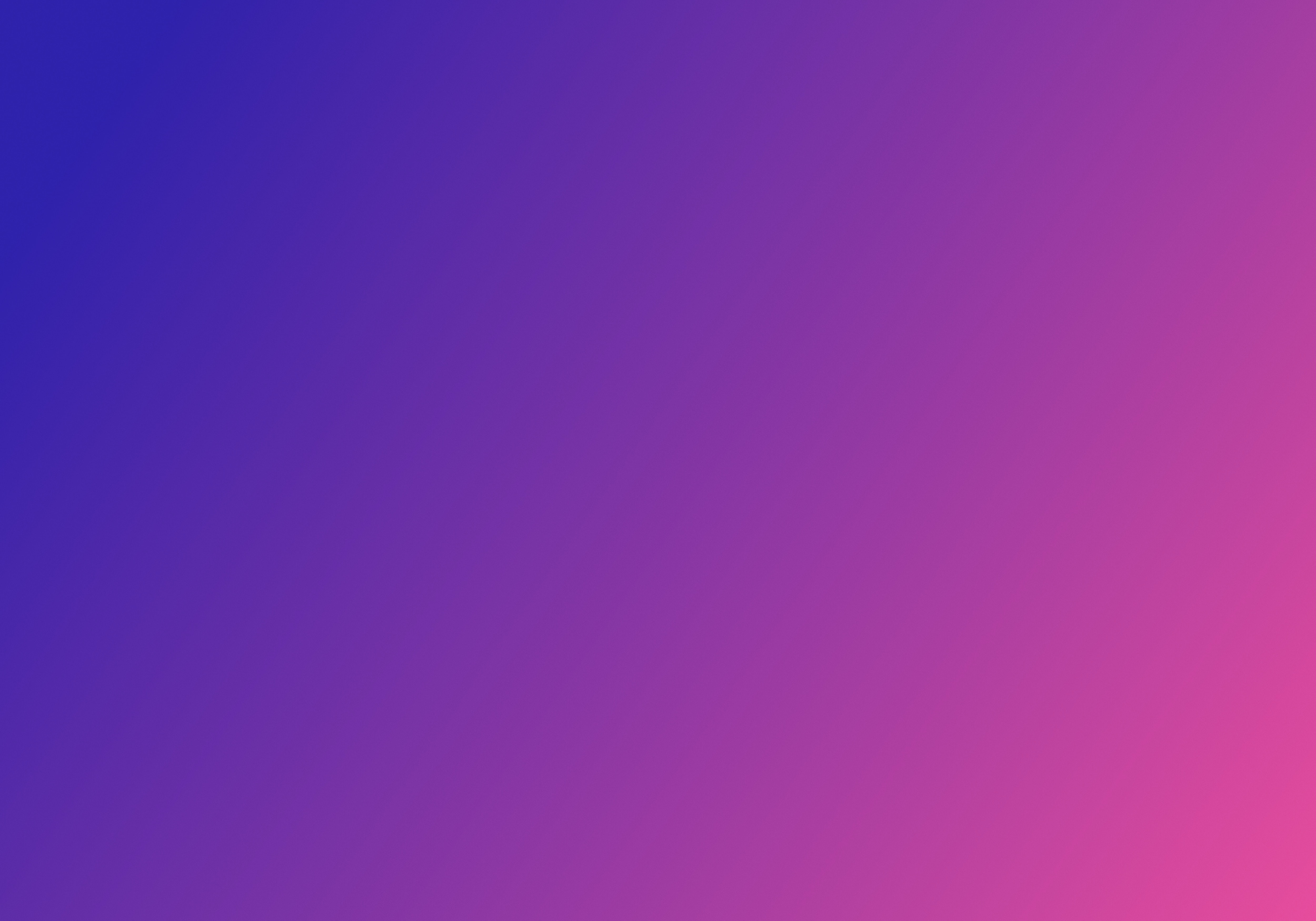
Enable the API
Before you can start making API calls, you'll need to activate the API in your Mautic dashboard. It’s a straightforward process:
(I'll explain further below on how to make your API access more robust and secure.)
Enable the API
First open the API configuration view: Go to Settings > Configuration > API Settings
in your Mautic dashboard.
Then find the API Enabled?
option and set it to Yes
. This turns on the API functionality. It enables the API for use via OAuth. (more on that below).
Do you plan to use your own scripts? Then you want to enable HTTP Basic Authentication (more on this below, too).
Set Enable HTTP basic auth?
to Yes
. In a nutshell: This allows you use the API with your username and password.
Primer on Authentication Methods
Mautic gives you two options when it comes to API authentication:
- HTTP Basic Authentication
- OAuth Authentication
I'll give you a brief overview over those to. This should allow you to decide which method you use.
HTTP Basic Authentication
HTTP Basic Auth is a straightforward method where you send a username and password with each request. This method is like logging into your dashboard every time you want to change something.
To use the API with HTTP Basic Auth, you'd simply log into the API with your username and password.
This method is great for internal applications but less secure because it transmits your credentials in each call.
I use HTTP Basic Auth for my own scripts, because it's simple to use.
OAuth Authentication
OAuth is more secure, but also more complex. It uses tokens instead of sending credentials repeatedly.
This method is preferred for applications exposed to the public or where security is a bigger concern.
I use this method when I try to connect a web service, like Zappier, to Mautic. The app handles the OAuth logic and I get more security.
But before we talk security, let's learn how to use the API.
Making Your First Requests
Now that you've enabled the API, let’s get down to business. Making your first API is easy. I’ll break it down into simple steps using cURL.
Open your Linux or Windows terminal/powershell.
For this to work I assume two things:
- You enabled HTTP Basic Auth.
- You're using your admin user for full system and API access.
If you see something like this, then your user doesn't have the roles/privileges to make this particular API call:
{"errors":[{"code":403,"message":"You do not have access to the requested area/action.","details":[]}]}
Fetching data via the API
In our first interaction with the API we'll fetch some data from Mautic. We'll make a GET request for that. The basic structure looks like this:
curl -u 'api_user:api_user_password' 'https://mautic.yoursite.com/api/${endpoint}/${identifier}?${queryParams}$'
Breakdown of the command
curl
: This is the command itself, used to transfer data to or from a server using various protocols, with HTTP being one of the most common.-u 'api_user:api_user_password'
: This option stands for "user". It is used to pass the username (api_user
) and password (api_user_password
) for HTTP Basic Authentication.- What follows is the API URL:
https://mautic.yoursite.com/api/${endpoint}/${identifier}
It has three components:- Base URL:
https://mautic.yoursite.com/api/
This is the (base) URL of your API. - Endpoint
${endpoint}
: The endpoint gives you access to an API functionality.contacts
for example lets you access your contact data. - Query Parameters: Query parameters let you filter data if you fetch data. They are optional.
- Base URL:
What Happens When You Run the Command?
When you execute this command, curl
makes a request to the specified URL. It includes the user credentials for authentication. The server at mautic.yoursite.com
will:
- process your request,
- authenticate using the provided credentials, and if successful,
- return the (filtered, if applicable) data from the endpoint.
Fetch real data.
Let's try to fetch real data—the very first user; the user with the ID 1. This should be your admin user that you created during the installation of Mautic.
curl -u 'api_user:api_user_password' 'https://mautic.yoursite.com/api/contacts/1'
curl [...] | ConvertFrom-Json | ConvertTo-Json
to your curl request. On Linux append (mind the dot):
curl [...] | jq .
If successful, the (really long) response will look similar to this (I shortened it):
{
"contact": {
"isPublished": true,
"createdBy": 1,
"createdByUser": "Tobias from Audienture",
"id": 1,
"fields": {
// very long and detailed list of all contact fields
"all": {
"id": "1",
"firstname": "Tobias",
"lastname": "from Audienture",
"email": "info@audienture.com",
// all contact fields in key: value format
}
},
"owner": null,
"ipAddresses": [
// ..
],
"tags": [
// ..
],
"utmtags": [
// ..
],
"stage": null,
"doNotContact": [],
"frequencyRules": [
// ..
]
}
}
If you get an error, you either don't have the right permissions or deleted the contact. Try another ID.
Let's try to add or change some data:
Use the API to create data
To create data you need to make a POST request to your API. You need to send a JSON body along with your cULR request. This body (also known as payload) contains the data you want to create.
curl -X POST -H "Content-Type: application/json" -u 'api:test123' 'https://mautic.mysit.com/api/contacts/new' -d '{"firstname": "John", "lastname": "Doe", "email": "john.doe@example.com"}'
-X POST
: This option specifies the HTTP method to use. In this case,-X
stands for "request method," andPOST
is the method used when you want to send data to the server. A POST request is often used for creating new resources or submitting form data.-H "Content-Type: application/json"
: This option adds a header to the HTTP request (-H
). The header"Content-Type: application/json"
tells the server that the body of the request is formatted as JSON. This is crucial as the API expects the data to be in JSON format.-d '{"firstname": "John", "lastname": "Doe", "email": "john.doe@example.com"}'
: The-d
flag stands for "data" and is used to include data to be sent with the request. Here,'{"key":"value"}'
represents the JSON-formatted data being sent to the server. This could be any JSON object, and in this example, it creates the contact with first name John, last name Doe and email john.doe@example.com.
Mautic's response to this request will be the newly created contact. I'll omit that.
What happens if john.doe@example.com
is already a contact?
The email field is a unique identifier. This means that there can only be one contact in your database that has the email address john.doe@example.com
.
Mautic will update the existing contact using the data you'll send in your POST request.
This means:
- Say,
{"firstname": "John", "lastname": "Doe", "email": "john.doe@example.com"}
exists in your database. - And you'll make a POST request to
/api/contacts/new
with the payload{"firstname": "Peter", "company": "ACME", "email": "john.doe@example.com"}
- Then Mautic will update the contact's first name from
John
toPeter
and add the company nameACME
. So, your contact's data will look like this:{"firstname": "Peter", "lastname": "Doe", "company": "ACME", "email": "john.doe@example.com"}
.
What happens if an error occurs?
If your data is malformed an error 400 occurs:
{
"errors": [
{
"message": "Looks like I encountered an error (error #400). If I do it again, please report me to the system administrator!",
"code": 400,
"type": null
}
]
}
Use the API to change data
If you want to change data, then your curl command stays almost the same. The "only" difference is the HTTP method used (and the data you sent with your API call, of course).
The HTTP method you'll use is either PATCH or PUT. Which you use depends on the behavior you expect from Mautic.
curl -X PATCH|PUT -u 'username:password' -H "Content-Type: application/json" -d '{"first_name": "Jane"}' "https://yourmauticinstance.com/api/contacts/123/edit"
Explanation of PATCH vs. PUT
This means PATCH doesn't create a new entity (e.g., a contact), PUT does. PATCH doesn't change data you haven't included in your API call, PUT does.
In the curl example above, PATCH would change the first name to Jane
, leaving the remaining fields of contact 123
untouched. PUT on the other hand would empty all fields of contact 123
and only set first name to Jane
, leaving you with an almost empty contact.
In more detail:
PATCH (click to expand)
You use PATCH for making (partial) updates to an existing resource (i.e., a contact, email, etc.).
PATCH will only update the data you send with your API call.
If you want to update the first name of a contact, then PATH will only update this contact field.
If the specified entity (a contact in this case) does not exist, Mautic should return a 404 error.
They way PATCH works:
- Mautic looks for the entity with the ID you sent via the API call.
- If the entity exists: Mautic updates the entity's properties using the data you provided.
- If the entity doesn't exist, yet: Mautic returns an error.
PUT (click to expand)
You use PUT for updating a resource completely or creating it if it doesn't exist.
When you make a PUT API call, you have to provide the full set of data, not just the parts you want to update. If you omit data that is already set, it gets deleted.
Say, you want to update a contact via PUT and omit the first name field, this means, the first name gets deleted.
If the entity (e.g., a contact) with the specified ID exists, it will be updated; if it does not exist, Mautic will create it.
They way PUT works:
- Mautic looks for the entity with the ID you sent via the API call.
- If the entity exists: Mautic clears all the data.
- If the entity doesn't exist, yet: Mautic creates a new entity.
- Mautic fills the entity with all the data you provided via the API call.
Choosing between PATCH and PUT depends on whether you expect the resource to exist and whether you intend to provide complete or partial data for updates.
Manage Security
Properly managing roles and permissions is important to make your Mautic API secure. You can use Mautic's role management system to achieve that:
When you access the Mautic API, the authenticated user's API access mirrors their user interface permissions.
How It Works
This means they can interact with the API endpoints exactly as they would within the Mautic UI, limited by their user role.
Let's assume you created a role called "Editor". This role is only permitted to create and edit their own emails.
A user with this role will only be able to access email-related endpoints in the API.
Simple, yet effective.
They won't be able to interact with emails someone else created. And they certainly couldn't modify your contact database, campaigns, or other elements outside their scope.
Basic Set-Up and Example
To minimize risks and ensure each API user can only access relevant data and functionalities, follow these steps to create narrowly scoped API roles:
- Navigate to the
Roles
section in your Mautic settings. - Create a new role specifically for API access. For instance, you might have a role named "API Access - Contacts Script" for a script that only needs to manage contact data.
- Carefully select the permissions for this role. Only grant access to the necessary features that the API needs to interact with.
- Once your role is configured, create a user assigned exclusively for API interactions.
- Go to the
Users
section and create a new user. Assign the user the role you just created, ensuring they are configured to use API access only. - Use strong, complex passwords for this user account, and consider changing passwords regularly to maintain security.
Let's assume you want to create/import users via a script. You create the "API Access - Contacts Script" role.
The most secure permission design for this is giving the API Access - Contacts Script role just the permission to create new contacts.
Now, even if your credentials leak, an attacker could "just" create new contacts. This might be a PITA, but your data is still secure. They could not read or modify or delete your contact database, campaigns or emails.
Handling Responses and Errors
Mautic will respond to your requests with JSON data. If your request is successful, you’ll get data related to your request, along with status codes like 200 OK
.
If something goes wrong, you might see 404 Not Found
or 500 Internal Server Error
.
Familiarize yourself with common error codes. If you see 400 Bad Request
, check your data formatting; 401 Unauthorized
means there’s an issue with your credentials or user's role permissions.
Libraries
Mautic provides a PHP library to make interacting with your API easier: GitHub - mautic/api-library: Mautic API Library.
We went through setting up and the ins and outs of using the Mautic API. You're well-equipped to get started with using the API.
You will have to experiment with different API functionalities to get a grasp of what is possible, but I am sure, you'll find something that makes your marketing life easier.
After all, using the API gives you a powerful way to access your data and automate scripts.
If you have any questions, please shoot me a message.
Happy marketing!
Comments ()